This Arduino tutorial shows how to interface the UNO board with ST7789 TFT display.
The ST7789 TFT module contains a display controller with the same name: ST7789. It’s a color display that uses SPI interface protocol and requires 3, 4 or 5 control pins, it’s low cost and easy to use. This display is an IPS display, it comes in different sizes (1.3″, 1.54″ …) but all of them should have the same resolution of 240×240 pixel, this means it has 57600 pixels. This module works with 3.3V only and it doesn’t support 5V (not 5V tolerant).
TFT: Thin-Film Transistor.
SPI: Serial Peripheral Interface.
IPS: In-Plane Switching.
The following image shows a ST7789 display module provided by Adafruit Industries:
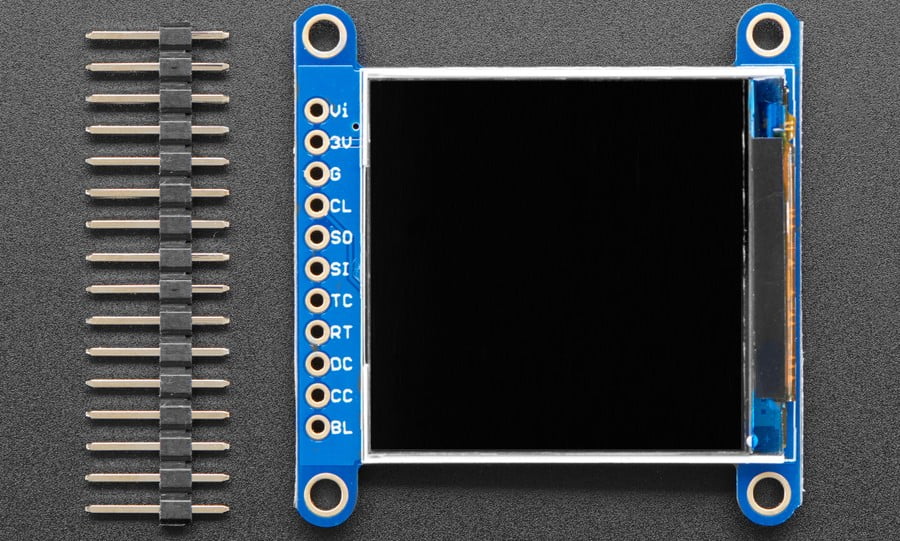
Another version of the ST7789 display module is shown below. This one has no CS (chip select) pin, its internally attached to GND:
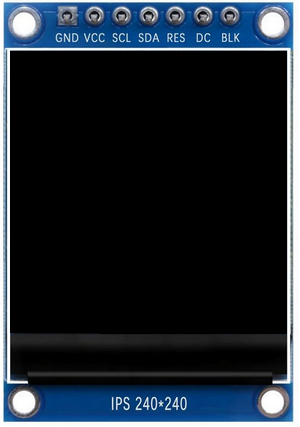
Project Hardware Required:
- Arduino UNO board —> ATmega328P datasheet
- ST7789 TFT display module (1.3″, 1.54″ …)
- 4 x 3.3k ohm resistor (+1 if the display module has CS pin)
- 4 x 2.2k ohm resistor (+1 if the display module has CS pin)
- Breadboard
- Jumper wires
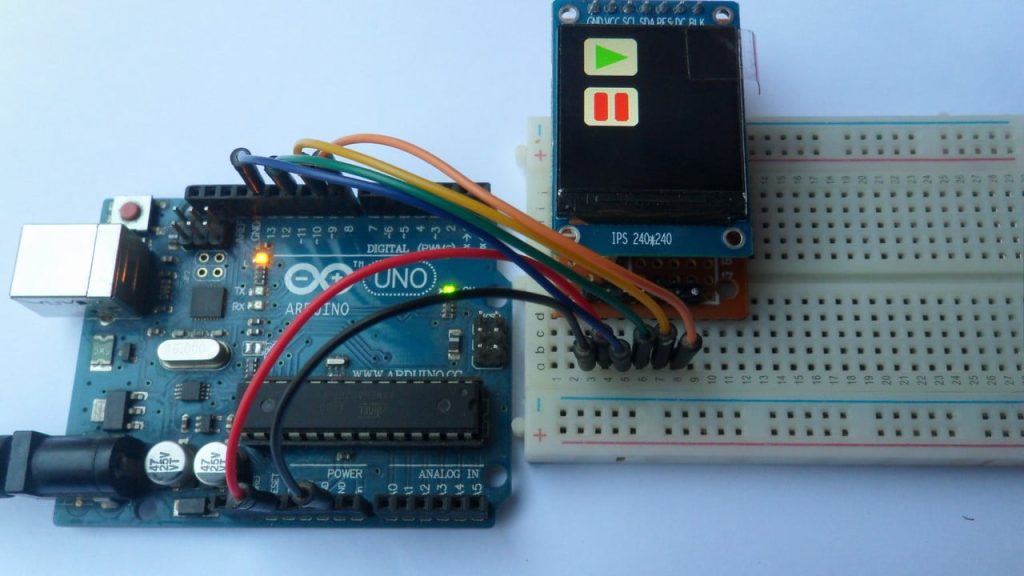
Interfacing Arduino UNO with ST7789 TFT circuit:
Project circuit schematic diagram is shown below.
The ST7789 display module shown in project circuit diagram has 7 pins: (from to left to right): GND (ground), VCC, SCL (serial clock), SDA (serial data), RES (reset), DC (or D/C: data/command) and BLK (back light).
Connecting the BLK pin is optional. The back light turns off when the BLK pin connected to the ground (GND).
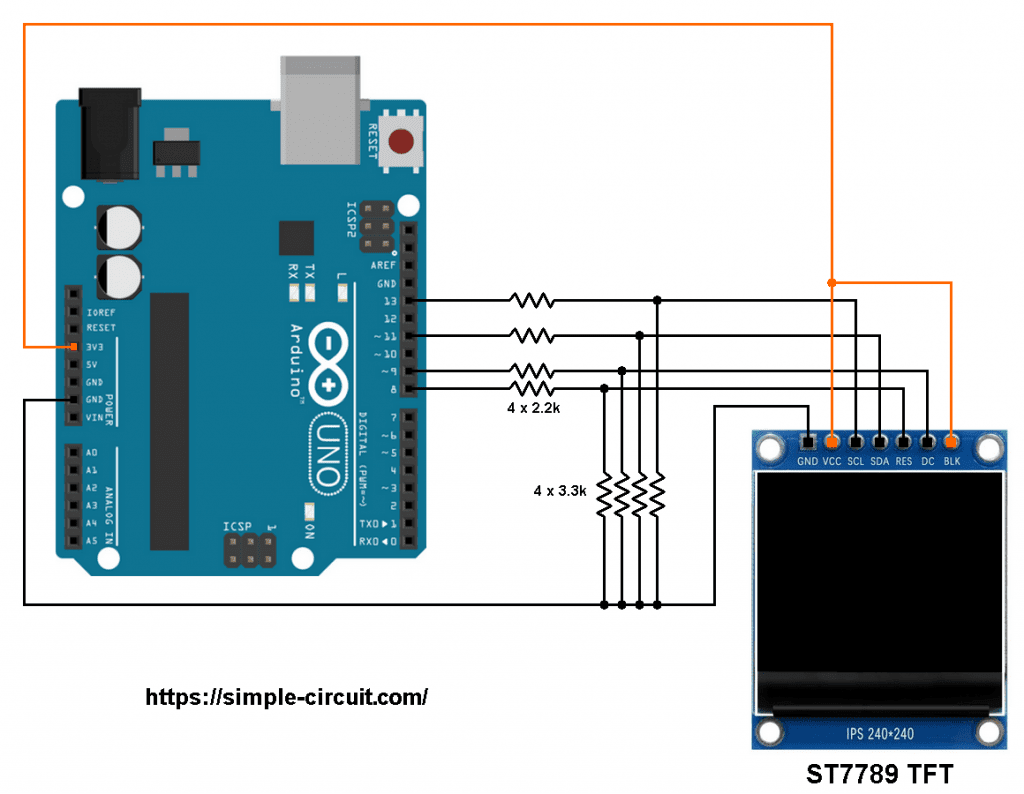
As mentioned above, the ST7789 TFT display controller works with 3.3V only (power supply and control lines). The display module is supplied with 3.3V (between VCC and GND) which comes from the Arduino board.
All Arduino UNO board output pins are 5V, connecting a 5V pin to the ST7789 TFT display may damage its controller.
To connect the Arduino to the display module, I used voltage divider for each line which means there are 4 voltage dividers. Each voltage divider consists of 2.2k and 3.3k resistors, this drops the 5V into 3V which is sufficient.
If the display module has a CS pin (Chip Select) then it should be connected to Arduino digital pin 10 through another voltage divider.
So, the ST7789 TFT display is connected to the Arduino board as follows (each one through voltage divider):
RST pin is connected to Arduino digital pin 8,
DC pin is connected to Arduino digital pin 9,
SDA pin is connected to Arduino digital pin 11,
SCL pin is connected to Arduino digital pin 13.
Other pins are connected as follows:
VCC pin is connected to Arduino 3V3 pin,
GND pin is connected to Arduino GND pin,
BL (LED) pin is connected to Arduino 3V3 pin (optional).
Interfacing Arduino UNO with ST7789 TFT code:
The following Arduino code requires two libraries from Adafruit Industries:
The first library is a driver for the ST7789 TFT display which can be installed from Arduino IDE library manager (Sketch —> Include Library —> Manage Libraries …, in the search box write “st7789” and install the one from Adafruit).
The second library is Adafruit graphics library which can be installed also from Arduino IDE library manager.
Both libraries can be installed manually, first download them from the following 2 links:
Adafruit ST7789 TFT library —-> direct link
Adafruit graphics library —-> direct link
After the download, go to Arduino IDE —> Sketch —> Include Library —> Add .ZIP Library … and browse for the .zip file (previously downloaded).
The same thing for the other library file.
Hints:
The 2 library files are included in the main code as shown below.
Including Arduino SPI library is optional!
1 2 3 | #include <Adafruit_GFX.h> // Core graphics library #include <Adafruit_ST7789.h> // Hardware-specific library for ST7789 #include <SPI.h> // Arduino SPI library |
The ST7789 TFT module pins (CS, RST and DC) connections are defined as shown below:
1 2 3 4 | // ST7789 TFT module connections #define TFT_CS 10 // define chip select pin #define TFT_DC 9 // define data/command pin #define TFT_RST 8 // define reset pin, or set to -1 and connect to Arduino RESET pin |
The other display pins (SDA and SCL) are connected to Arduino hardware SPI pins (digital pin 11 and digital pin 13).
The Adafruit ST7789 library is initialized with this line:
1 2 | // Initialize Adafruit ST7789 TFT library Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST); |
And the TFT display is initialized using the following command:
1 2 | // if the display has CS pin try with SPI_MODE0 tft.init(240, 240, SPI_MODE2); // Init ST7789 display 240x240 pixel |
The display may not work if it has a CS pin, try with SPI_MODE0 which is the default mode of the library or just use: tft.init(240, 240);
Rest of code is described through comments.
Full Arduino code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 | /* * Interfacing Arduino with ST7789 TFT display (240x240 pixel). * Graphics test example. * This is a free software with NO WARRANTY. * http://simple-circuit.com/ */ /************************************************************************** This is a library for several Adafruit displays based on ST77* drivers. Works with the Adafruit 1.8" TFT Breakout w/SD card ----> http://www.adafruit.com/products/358 The 1.8" TFT shield ----> https://www.adafruit.com/product/802 The 1.44" TFT breakout ----> https://www.adafruit.com/product/2088 as well as Adafruit raw 1.8" TFT display ----> http://www.adafruit.com/products/618 Check out the links above for our tutorials and wiring diagrams. These displays use SPI to communicate, 4 or 5 pins are required to interface (RST is optional). Adafruit invests time and resources providing this open source code, please support Adafruit and open-source hardware by purchasing products from Adafruit! Written by Limor Fried/Ladyada for Adafruit Industries. MIT license, all text above must be included in any redistribution **************************************************************************/ #include <Adafruit_GFX.h> // Core graphics library #include <Adafruit_ST7789.h> // Hardware-specific library for ST7789 #include <SPI.h> // Arduino SPI library // ST7789 TFT module connections #define TFT_CS 10 // define chip select pin #define TFT_DC 9 // define data/command pin #define TFT_RST 8 // define reset pin, or set to -1 and connect to Arduino RESET pin // Initialize Adafruit ST7789 TFT library Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST); float p = 3.1415926; void setup(void) { Serial.begin(9600); Serial.print(F("Hello! ST77xx TFT Test")); // if the display has CS pin try with SPI_MODE0 tft.init(240, 240, SPI_MODE2); // Init ST7789 display 240x240 pixel // if the screen is flipped, remove this command tft.setRotation(2); Serial.println(F("Initialized")); uint16_t time = millis(); tft.fillScreen(ST77XX_BLACK); time = millis() - time; Serial.println(time, DEC); delay(500); // large block of text tft.fillScreen(ST77XX_BLACK); testdrawtext("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur adipiscing ante sed nibh tincidunt feugiat. Maecenas enim massa, fringilla sed malesuada et, malesuada sit amet turpis. Sed porttitor neque ut ante pretium vitae malesuada nunc bibendum. Nullam aliquet ultrices massa eu hendrerit. Ut sed nisi lorem. In vestibulum purus a tortor imperdiet posuere. ", ST77XX_WHITE); delay(1000); // tft print function! tftPrintTest(); delay(4000); // a single pixel tft.drawPixel(tft.width()/2, tft.height()/2, ST77XX_GREEN); delay(500); // line draw test testlines(ST77XX_YELLOW); delay(500); // optimized lines testfastlines(ST77XX_RED, ST77XX_BLUE); delay(500); testdrawrects(ST77XX_GREEN); delay(500); testfillrects(ST77XX_YELLOW, ST77XX_MAGENTA); delay(500); tft.fillScreen(ST77XX_BLACK); testfillcircles(10, ST77XX_BLUE); testdrawcircles(10, ST77XX_WHITE); delay(500); testroundrects(); delay(500); testtriangles(); delay(500); mediabuttons(); delay(500); Serial.println("done"); delay(1000); } void loop() { tft.invertDisplay(true); delay(500); tft.invertDisplay(false); delay(500); } void testlines(uint16_t color) { tft.fillScreen(ST77XX_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(0, 0, x, tft.height()-1, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(0, 0, tft.width()-1, y, color); delay(0); } tft.fillScreen(ST77XX_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(tft.width()-1, 0, x, tft.height()-1, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(tft.width()-1, 0, 0, y, color); delay(0); } tft.fillScreen(ST77XX_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(0, tft.height()-1, x, 0, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(0, tft.height()-1, tft.width()-1, y, color); delay(0); } tft.fillScreen(ST77XX_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawLine(tft.width()-1, tft.height()-1, x, 0, color); delay(0); } for (int16_t y=0; y < tft.height(); y+=6) { tft.drawLine(tft.width()-1, tft.height()-1, 0, y, color); delay(0); } } void testdrawtext(char *text, uint16_t color) { tft.setCursor(0, 0); tft.setTextColor(color); tft.setTextWrap(true); tft.print(text); } void testfastlines(uint16_t color1, uint16_t color2) { tft.fillScreen(ST77XX_BLACK); for (int16_t y=0; y < tft.height(); y+=5) { tft.drawFastHLine(0, y, tft.width(), color1); } for (int16_t x=0; x < tft.width(); x+=5) { tft.drawFastVLine(x, 0, tft.height(), color2); } } void testdrawrects(uint16_t color) { tft.fillScreen(ST77XX_BLACK); for (int16_t x=0; x < tft.width(); x+=6) { tft.drawRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color); } } void testfillrects(uint16_t color1, uint16_t color2) { tft.fillScreen(ST77XX_BLACK); for (int16_t x=tft.width()-1; x > 6; x-=6) { tft.fillRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color1); tft.drawRect(tft.width()/2 -x/2, tft.height()/2 -x/2 , x, x, color2); } } void testfillcircles(uint8_t radius, uint16_t color) { for (int16_t x=radius; x < tft.width(); x+=radius*2) { for (int16_t y=radius; y < tft.height(); y+=radius*2) { tft.fillCircle(x, y, radius, color); } } } void testdrawcircles(uint8_t radius, uint16_t color) { for (int16_t x=0; x < tft.width()+radius; x+=radius*2) { for (int16_t y=0; y < tft.height()+radius; y+=radius*2) { tft.drawCircle(x, y, radius, color); } } } void testtriangles() { tft.fillScreen(ST77XX_BLACK); int color = 0xF800; int t; int w = tft.width()/2; int x = tft.height()-1; int y = 0; int z = tft.width(); for(t = 0 ; t <= 15; t++) { tft.drawTriangle(w, y, y, x, z, x, color); x-=4; y+=4; z-=4; color+=100; } } void testroundrects() { tft.fillScreen(ST77XX_BLACK); int color = 100; int i; int t; for(t = 0 ; t <= 4; t+=1) { int x = 0; int y = 0; int w = tft.width()-2; int h = tft.height()-2; for(i = 0 ; i <= 16; i+=1) { tft.drawRoundRect(x, y, w, h, 5, color); x+=2; y+=3; w-=4; h-=6; color+=1100; } color+=100; } } void tftPrintTest() { tft.setTextWrap(false); tft.fillScreen(ST77XX_BLACK); tft.setCursor(0, 30); tft.setTextColor(ST77XX_RED); tft.setTextSize(1); tft.println("Hello World!"); tft.setTextColor(ST77XX_YELLOW); tft.setTextSize(2); tft.println("Hello World!"); tft.setTextColor(ST77XX_GREEN); tft.setTextSize(3); tft.println("Hello World!"); tft.setTextColor(ST77XX_BLUE); tft.setTextSize(4); tft.print(1234.567); delay(1500); tft.setCursor(0, 0); tft.fillScreen(ST77XX_BLACK); tft.setTextColor(ST77XX_WHITE); tft.setTextSize(0); tft.println("Hello World!"); tft.setTextSize(1); tft.setTextColor(ST77XX_GREEN); tft.print(p, 6); tft.println(" Want pi?"); tft.println(" "); tft.print(8675309, HEX); // print 8,675,309 out in HEX! tft.println(" Print HEX!"); tft.println(" "); tft.setTextColor(ST77XX_WHITE); tft.println("Sketch has been"); tft.println("running for: "); tft.setTextColor(ST77XX_MAGENTA); tft.print(millis() / 1000); tft.setTextColor(ST77XX_WHITE); tft.print(" seconds."); } void mediabuttons() { // play tft.fillScreen(ST77XX_BLACK); tft.fillRoundRect(25, 10, 78, 60, 8, ST77XX_WHITE); tft.fillTriangle(42, 20, 42, 60, 90, 40, ST77XX_RED); delay(500); // pause tft.fillRoundRect(25, 90, 78, 60, 8, ST77XX_WHITE); tft.fillRoundRect(39, 98, 20, 45, 5, ST77XX_GREEN); tft.fillRoundRect(69, 98, 20, 45, 5, ST77XX_GREEN); delay(500); // play color tft.fillTriangle(42, 20, 42, 60, 90, 40, ST77XX_BLUE); delay(50); // pause color tft.fillRoundRect(39, 98, 20, 45, 5, ST77XX_RED); tft.fillRoundRect(69, 98, 20, 45, 5, ST77XX_RED); // play color tft.fillTriangle(42, 20, 42, 60, 90, 40, ST77XX_GREEN); } |
The video below shows my breadboard test circuit:
Related Project:
Arduino Interface with ST7789 Color TFT Display
Discover more from Simple Circuit
Subscribe to get the latest posts sent to your email.
HELP
C:\Users\sefao\AppData\Local\Temp\.arduinoIDE-unsaved202325-17488-1g2uwtf.gqif\sketch_mar5b\sketch_mar5b.ino:16:1: error: ‘tft’ does not name a type
tft.init(240, 240, SPI_MODE3); // Init ST7789 display 240×240 pixel
^~~
C:\Users\sefao\AppData\Local\Temp\.arduinoIDE-unsaved202325-17488-1g2uwtf.gqif\sketch_mar5b\sketch_mar5b.ino:58:17: error: redefinition of ‘Adafruit_ST7789 tft’
Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST);
^~~
C:\Users\sefao\AppData\Local\Temp\.arduinoIDE-unsaved202325-17488-1g2uwtf.gqif\sketch_mar5b\sketch_mar5b.ino:13:17: note: ‘Adafruit_ST7789 tft’ previously declared here
Adafruit_ST7789 tft = Adafruit_ST7789(TFT_CS, TFT_DC, TFT_RST);
^~~
C:\Users\sefao\AppData\Local\Temp\.arduinoIDE-unsaved202325-17488-1g2uwtf.gqif\sketch_mar5b\sketch_mar5b.ino: In function ‘void mediabuttons()’:
C:\Users\sefao\AppData\Local\Temp\.arduinoIDE-unsaved202325-17488-1g2uwtf.gqif\sketch_mar5b\sketch_mar5b.ino:314:12: error: expected ‘}’ at end of input
delay(50);
^
exit status 1
Compilation error: ‘tft’ does not name a type
WHAT CAN I DO FOR THAT?????
Hello, help me pls.
Why is the voltage divider necessary for the control pins? (SCL, SDA, RST, DC)
In the videos that I was watching they do not connect any type of resistance, they do it directly to the arduino UNO.
However, I am having problems with my display. the model is zjy-ips130-v2.0
I use the code and everything loads fine, but I see that the display comes out very dark and each pixel seems to have an error, it’s twinkling…
but when I press the Reset button on the arduino, or reload the program, in those 1-2 seconds the screen shows correctly what it should show initially, is it a problem of voltage to the pins then?
As described above, the display works with 3.3V only and the Arduino outputs are 5V, therefore we need to decrease the Arduino 5V into 3.3V and for this reason we used the voltage divider resistors.
In the video the voltage divider resistors are already used and they are soldered in the DIY PCB (may be not shown clearly). If you’ve an Arduino UNO or similar board (any 5V board) then you must use the voltage divider resistors!
Sebebini bulabildiniz mi bende aynı şekilde bu durumla karşı karşıya kaldım bağlantılarım doğru ekran karanlık ardiuno reset düğmesine bağladığım zaman ekran parlaklığı geliyor geri karanlık oluyor
Hi! I’m having some trouble while uploading the code to my Arduino Uno.
Arduino:1.8.11 Hourly Build 2019/12/30 10:12 (Mac OS X), Tarjeta:”Arduino Uno”
In file included from /Users/lesu/Documents/Arduino/libraries/Adafruit_ST7735_and_ST7789_Library-1.5.13/Adafruit_ST7789.h:4:0,
from /Users/lesu/Documents/Arduino/Display_ST7789/Display_ST7789.ino:2:
/Users/lesu/Documents/Arduino/libraries/Adafruit_ST7735_and_ST7789_Library-1.5.13/Adafruit_ST77xx.h:31:10: fatal error: Adafruit_SPITFT.h: No such file or directory
#include
^~~~~~~~~~~~~~~~~~~
compilation terminated.
exit status 1
Do you know what this means? I’ve tried deleting the libraries and installing them but that doesn’t seem to solve my problem.
Also, I’d like to ask is there any change if instead of using resistors as suggested I use a level converter por the digital inputs?
https://es.aliexpress.com/wholesale?catId=0&initiative_id=&origin=y&SearchText=arduino+level+converter
Thanks for your help!!
Hi again, finally I could solve it by my own. I’ll leave what I did in case someone else has the same problem.
Basically when I downloaded the libraries I chose the option “Download all Adafruit libraries”. Apparently one of the other libraries was causing trouble. To solve it I deleted the libraries following the steps described in this page https://forum.arduino.cc/index.php?topic=295275.0
Once I did that, I installed only the libraries that are recommended in this tutorial and the compiling was perfect.
Finally, I used a level converter that let me decrease the voltage without using resistors!
check for missing file Adafruit_SPITFT.h: No such file or directory
You get only around 2 volts through the voltage divider, isnt that a little too low?
Use voltage divider formula and you’ll get 3 Volts (not 2 Volts):
Vout (goes to ST7789 TFT) = 5V (from Arduino output pin) x 3.3k/(3.3k + 2.2k) = 5 x 3.3/5.5 = 3 volts.
Some microcontrollers output high 3.3 instead of 5. This voltage divider gives 2,2 if so and yes probably be too low to work. Set a pin high and check without voltage divider. If voltage >3 and <3.3 you will not need to use a voltage divider. It might not be a bad idea to just remove the 3.3K resistor and let the 2.2K resistor cut down the current.
That’s what i finaly thought, you just confirm to me i had did a terrible newbie mistake ;-( After following all your instruction screen was working fine for months.. But backlight was too strong for me during night, so i wanted to try connect BLK to GROUND as you suggere…. But i had your setup with BLK connect to vcc 3.3v, what make me confused and did not think about switching with ground (maybe should be good to precise for others newbees like me).. So i thought maybe there is some protection inside arduino or some funcion i ignore.. But no !! you confirm to me i did a short circuit ;-)). I burn the TFT, and after check i burned some pins of arduino too, and my relay module ;-((
The good news of this story is i had learned of my mistake and never do it again 😉 and in 1 month i receive the new screen for my clock alarm v2 😉
So thank you.. It’s not easy to find information about thoses screen and BLK funccion.. Can we use a potentiometer to ajust luminosity ? (The strange things with my short circuit story is that TFT funccion display burnout but no the led, i still have light).
I saw a man using tft reset pin : pinmode(reset,output);
analogWrite(reset,true), delay(200);analogwrite(reset,false) for software simulate of BLK, i try it but it only switch off the display, some light was still there. So i wanted to try something with analogwrite to send 3,3v or 0v (i did not found any information about that)..
Do you know what the reset is use for ? Just reset display i suppose…
So thanks again, you are the only one to have such a good and almost full explanation with how to use thoses screen..
Nicolas from france !
After long time this working for me, i wanted to use BLK, so i connect it to Ground… and my screen is dead now (but not sure it’s the cause).. but now something make me confuse, you connect BLK to GND, so it means connect GND direct with 3.3v on arduino (BLK is connected to 3.3v), how can’t it damage it ?
Thank’s for your light !!! And for this perfect tutorial..
The BLK pin is just for the back light LED. If it is connected to VCC (+3.3V) or left unconnected the backlight will be ON and if it is connected to GND the backlight will be OFF. You cannot connect it to VCC and GND at the same time because you will do a short circuit and even your Arduino board may be damaged!
Connecting the display to 5V may damage it (power supply or data lines), that’s why the voltage dividers are used!
Also reversing the polarity of the 3.3V power supply may damage it.
This code was not working with my display from china, because without CS you need to use SPI_MODE3
So, display initializing command becomes (line: 50):
tft.init(240, 240, SPI_MODE3);
This may help others, thank you!
For colour issue in china display you need to change spi mode in two places
Adafruit_ST77xx.h
//spiMode = SPI_MODE0; ///< Certain display needs MODE3 instead
spiMode = SPI_MODE3; ///< Certain display needs MODE3 instead
Adafruit_ST7789.h
void init(uint16_t width, uint16_t height, uint8_t spiMode = SPI_MODE3);
i faced colour issue, that display purchased in aliexpress
after long studies i done above modification
now works
Just tested today (24/12/2019)
Instead of this modification you may just set SPI mode in the main code, for example:
tft.init(240, 240, SPI_MODE3);
Can you give us a picture of your display (or Aliexpress link)?
https://www.aliexpress.com/item/32969298268.html
i tried as you told but not works
after two weeks of analyzing i done the modification
In the Aliexpress link I’m seeing 2 types: 0.96″ and 1.3″.
Which one did you buy please?
1.3″
It looks the same as the one which I’m using!
Thank you
Hi friends
i am unlucky to use this display, i think so.
the same program i used and removed all content like draw lines, circles, like all by one by one the display works in all stage. finally when removing testroundrectangle(); function the display gone half.
if i am using this in the program display works good
so i included the function to execute first, like when power on test round rectangle will come then my project content will comes net. like power up screen
then i decided to copy the program from following link
https://simple-circuit.com/tft-st7789-arduino-dht11-sensor/
directly i uploaded but the display direction is not good
same parameters used in the program like spimode 3 setrotation 2 etc but different result
kindly suggest me some idea still my project is in first phase
This code _as it is_ should work perfectly and without any problem!
Try with the following:
Update Adafruit ST7735/ST7789 library (current version 1.5.9),
Update Adafruit graphics library (current version 1.7.3),
You can update both libraries using Arduino IDE library manager (Tools —> Manage Libraries…).
And try using the latest version of Arduino IDE (current version 1.8.10).
Thanks friend,
i am new to Arduino and really happy you all reply immediately.
really works after update. its miracle
one program working on old file another working in new one. now i tested both program after update working fine
Just one thing, you should use Mode 3 for display without CS.
SPI_MODE3
thanks that’s help me…
Thank’s, you save my day
Hi, I have a very similar tft display, but it has a cs pin. What do i do with it?
Connect it to Arduino pin 10 through voltage divider (2.2k and 3.3k resistors).
Hello, i tested it with Arduino Micro Pro. As is described, it was not working. After change Res to pin 9, DC to pin 8, BLK to pin 10 is now working nice. Thank for inspiration.
I cannot connect mine to the arduino as it has come with a white plug with 8 wires , i cant find a pinout drawing to let me connect
Hello, how can I connect this display to raspberry pi zero?